Having recently gotten beginner-level-comfortable with the Zibo 737-800 in X-Plane, I fired up Vatsim to take to the virtual skies to see if I could manage my way from Raleigh-Durham International Airport (KRDU) to Charlotte/Douglas International Airport (KCLT) successfully. It can be a little daunting with the addition of live Air Traffic Control + other traffic, but really creates a greater sense of realism. I’m not very fluid currently, but hope to do this more and improve as time goes on.
As for the purpose of this post, it’s one of those things I’m not quite sure anyone else will get any use out of, but wanted to share regardless. While getting prepped to fly last night, I went over to Simbrief to create my flightplan. After this, I downloaded it in X-Plane 11/12 format and dropped it into my X-Plane “FMS plans” directory so that I could load it up in the Zibo’s FMC. Then, I proceeded to use the handy feature to send the plan directly to the Vatsim servers and file it accordingly.
This process is not too bad, but I like automating things where I can and stumbled accross a page on Navigraph Developers describing how to retrieve your latest Simbrief-created flight plan in XML format. After taking a look at the XML, I figured I could script something up to make it so that I could create the plan on Simbrief, run the script, and get flying (more or less) vs. having to manually download things and place them into certain directories.
Script Overview
In a nutshell, this script (meant to run on Linux) hits a Simbrief endpoint that provides the most recently created flight plan for a given Simbrief Pilot ID or username. It does a bit of parsing of the XML file to download the plan in X-Plane 11/12 format and place it into the correct X-Plane directory. It can also use SMB mounts for in the event you want to drop the plan onto a Windows system running X-Plane. Outside of that, the flight plan overview will be produced in the terminal as well as the proper link to file your plan with Vatsim.
Please feel free to use and modify at will! If the comments do not explain something well enough, reach out!
Note: To be clear, this will ONLY pull the flight you last generated on Simbrief (ie: you cannot specify one of your previously saved flights).
The Script
#!/bin/bash
####################################
# This script enables you to pull #
# the latest X-Plane 11/12 flight #
# plan file from Simbrief based #
# your Simbrief Pilot ID and place #
# it into the proper directory for #
# loading in X-Plane #
####################################
usage() { echo "USAGE: $0
General Options:
-f Path to X-Plane \"FMS plans\" directory
-n Optional file plan name (Default: SB.fms)
-p Simbrief Pilot ID
-w Working directory
-v List all current variable values
SMB Mount Options (all required to be set when using smb):
-d Target share name on system running X-Plane (Default: "FMS plans")
-h Hostname or IP address of smb target system running X-Plane
-l Local mount directory name if using smb (Default: "mountdir")
-s Use smb for remotely mounting X-Plane "FMS plans" directory (true or false) (Default: false)" 1>&2; exit 1; }
showvars() { echo "======================VARIABLE READOUT======================
Simbrief Pilot ID: $SB_PILOT_ID
Working Directory: $WORKING_DIR
FMS Plans Directory: $XPLANE_FMS_DIR
Flight Plan Output Name: $XPLANE_FP_NAME
Use SMB Mount: $USE_SMB
Remote Host: $SMB_HOST
Remote Share Name: $SMB_FMS_SHARE_NAME
Local Mount Directory: $LOCAL_MOUNT_DIR_NAME
============================================================" 1>&2; exit 1; }
## User Variables - Note: You can hardcode any variables you wish in order not to have to input them as execution options
SB_PILOT_ID= # Your Simbrief Pilot ID found in account settings (https://dispatch.simbrief.com/account). Can also be set with -p option
WORKING_DIR= # Directory to work from (you create this). Can also be set with -w option
XPLANE_FMS_DIR= # Path to your X-Plane "FMS plans" directory (X-Plane 11 directory -> Output -> FMS plans). Can also be set with -f option
XPLANE_FP_NAME= SB.fms # Change this if a different filename in your "FMS plans" directory is desired. Can also be set with -n option
## Below variables apply to mounting X-Plane "FMS plans" directory remotely via smb. The expectation is that X-Plane's "FMS plans" directory is shared with
## that name and is accessible & writable by guest without a password. You can also change the mount parameters below if you want to use a specifc user,
## provide a password, change the mount path, etc.
USE_SMB=false # Set to "true" if using smb. Can also be set with -s option
SMB_HOST= # Hostname or IP of Windows target. Can also be set with -h option
SMB_FMS_SHARE_NAME="FMS plans" # Windows share name. Can also be set with -s option
LOCAL_MOUNT_DIR_NAME=mountdir # This directory will be created for the smb mount. Can also be set with the -l option
while getopts ":p:w:f:n:l:s:h:d:v" opt
do
case $opt in
p) SB_PILOT_ID=$OPTARG;;
w) WORKING_DIR=$OPTARG;;
f) XPLANE_FMS_DIR=$OPTARG;;
n) XPLANE_FP_NAME=$OPTARG;;
l) LOCAL_MOUNT_DIR_NAME=$OPTARG;;
s) USE_SMB=$OPTARG;;
h) SMB_HOST=$OPTARG;;
d) SMB_FMS_SHARE_NAME=$OPTARG;;
v) showvars;;
*) usage;;
esac
done
## Show variable summary
echo -e "\n======================VARIABLE READOUT======================"
echo -e "Simbrief Pilot ID: $SB_PILOT_ID"
echo "Working Directory: $WORKING_DIR"
echo "FMS Plans Directory: $XPLANE_FMS_DIR"
echo "Flight Plan Output Name: $XPLANE_FP_NAME"
echo "Use SMB Mount: $USE_SMB"
if [ $USE_SMB == true ]
then
echo "Remote Host: $SMB_HOST"
echo "Remote Share Name: $SMB_FMS_SHARE_NAME"
echo -e "Local Mount Directory: $LOCAL_MOUNT_DIR_NAME"
fi
echo -e "============================================================\n"
## Fetch latest flight plan XML from Simbrief and form URL to download
SB_FP_XML_URL=https://www.simbrief.com/api/xml.fetcher.php?userid=$SB_PILOT_ID
SB_FP_XPE_FILENAME=$(curl --silent $SB_FP_XML_URL | grep xml_file | awk -F"/" '{print $7}' | tr -d \< | sed s/XML/XPE/ | sed s/.xml/.fms/)
SB_FP_XPE_URL=https://www.simbrief.com/ofp/flightplans/$SB_FP_XPE_FILENAME
## Safety check for working directory
if [ ! -d "$WORKING_DIR" ]
then
echo $(usage)
echo -e "\nWorking directory not found. Please verify WORKING_DIR variable is correct.\n"
exit
fi
## If using smb, check to make sure all necessary variables are populated
if [[ -z "$SMB_HOST" || -z "$LOCAL_MOUNT_DIR_NAME" || -z "$SMB_FMS_SHARE_NAME" ]]
then
echo $(usage)
echo -e "\n Required smb variables unset. Please set all required smb variables and try again.\n"
exit
fi
## If using smb, make mount directory if necessary and mount FMS plans share
if [[ $USE_SMB == true ]]
then
mkdir -p $WORKING_DIR/$LOCAL_MOUNT_DIR_NAME
mount.cifs //$SMB_HOST/"$SMB_FMS_SHARE_NAME" $WORKING_DIR/$LOCAL_MOUNT_DIR_NAME -o user=guest,password=''
fi
## Safety check for flight plan directory
if [ ! -d "$XPLANE_FMS_DIR" ]
then
echo $(usage)
echo -e "\nFlight plan directory not found. Please verify XPLANE_FMS_DIR variable is correct.\n"
if [[ $USE_SMB == true ]]
then
umount -q $WORKING_DIR/$LOCAL_MOUNT_DIR_NAME
rmdir "$WORKING_DIR/$LOCAL_MOUNT_DIR_NAME"
fi
exit
fi
## Download latest flight plan in X-Plane 11/12 format and place into "FMS plans" directory
echo -e "Retrieving flight plan and placing it into $XPLANE_FMS_DIR\n"
echo "================WGET OUTPUT================"
wget --no-verbose $SB_FP_XPE_URL -O $XPLANE_FMS_DIR/$XPLANE_FP_NAME
echo "==========================================="
## Display overview
echo -e "\n==============FLIGHT OVERVIEW=============="
curl --silent $SB_FP_XML_URL | sed -n "/<general>/,/<\/general>/p" | sed "s/ <//g" | sed 's/^[ \t]*//' | sed 's/>/: /g' | grep -v general | cut -d"<" -f1 | sed 's/./\u&/'
echo -e "============================================"
## Output link to file with Vatsim
echo -e "\nShould you wish to file this flight plan with Vatsim, use the following link:"
curl --silent $SB_FP_XML_URL | grep "<link>https://my.vatsim.net/pilots/flightplan" | sed 's/<[^>]*>/\n/g'
## Cleanup for smb mount
if [[ $USE_SMB == true ]]
then
umount -q $WORKING_DIR/$LOCAL_MOUNT_DIR_NAME
rmdir $WORKING_DIR/$LOCAL_MOUNT_DIR_NAME
fi
exit
Examples/Expected Output
Viewing Current Variables
# ./fetch_simbrief.sh -v
======================VARIABLE READOUT======================
Simbrief Pilot ID: 123456
Working Directory: /root/xplane_scripts/fetch_simbrief
FMS Plans Directory: /root/xplane_scripts/fetch_simbrief/mountdir
Flight Plan Output Name: SB.fms
Use SMB Mount: false
Remote Host: windowsbox
Remote Share Name: FMS plans
Local Mount Directory: mountdir
============================================================
Locally (no SMB)
# ./fetch_simbrief.sh -w /root/xplane_scripts/fetch_simbrief -f /root/xplane_scripts/fetch_simbrief/fms
======================VARIABLE READOUT======================
Simbrief Pilot ID: 123456
Working Directory: /root/xplane_scripts/fetch_simbrief
FMS Plans Directory: /root/xplane_scripts/fetch_simbrief/fms
Flight Plan Output Name: SB.fms
Use SMB Mount: false
============================================================
Retrieving flight plan and placing it into /root/xplane_scripts/fetch_simbrief/fms
================WGET OUTPUT================
2024-01-13 16:36:32 URL:https://www.simbrief.com/system/briefing.fmsdl.php?formatget=flightplans/KRDUKCLT_XPE_1705153423.fms [320/320] -> "/root/xplane_scripts/fetch_simbrief/fms/SB.fms" [1]
===========================================
==============FLIGHT OVERVIEW==============
Release: 2
Icao_airline: AAL
Flight_number: 2964
Is_etops: 0
Dx_rmk: PLANNED OPTIMUM FLIGHT LEVEL
Sys_rmk/:
Is_detailed_profile: 1
Cruise_profile: CI 78
Climb_profile: 250/280/78
Descent_profile: 78/280/250
Alternate_profile: CI0
Reserve_profile: RES
Costindex: 78
Cont_rule: 15 MIN
Initial_altitude: 22000
Stepclimb_string: KRDU/0220
Avg_temp_dev: 6
Avg_tropopause: 50196
Avg_wind_comp: -89
Avg_wind_dir: 240
Avg_wind_spd: 103
Gc_distance: 113
Route_distance: 167
Air_distance: 235
Total_burn: 4869
Cruise_tas: 443
Cruise_mach: .72
Passengers: 183
Route: SHPRD4 CATAR DCT SDAIL CHSLY5
Route_ifps: SHPRD4 CATAR DCT SDAIL CHSLY5
Route_navigraph: SHPRD4 CATAR DCT SDAIL CHSLY5
============================================
Should you wish to file this flight plan with Vatsim, use the following link:
https://my.vatsim.net/pilots/flightplan?raw=%28FPL-AAL2964-IS+-B738%2FM-SDE2E3FGHIRWXY%2FLB1+-KRDU1048+-N0443F220+SHPRD4+CATAR+SDAIL+CHSLY5+-KCLT0045+KATL+-PBN%2FA1B1C1D1S1S2+DOF%2F240113+REG%2FN806SB+EET%2FKZTL0017+OPR%2FAAL+PER%2FC+RMK%2FTCAS+SIMBRIEF%29&fuel_time=0225
Remote (with SMB)
# ./fetch_simbrief.sh -w /root/xplane_scripts/fetch_simbrief -f /root/xplane_scripts/fetch_simbrief/mountdir -s true
======================VARIABLE READOUT======================
Simbrief Pilot ID: 123456
Working Directory: /root/xplane_scripts/fetch_simbrief
FMS Plans Directory: /root/xplane_scripts/fetch_simbrief/mountdir
Flight Plan Output Name: SB.fms
Use SMB Mount: true
Remote Host: windowsbox
Remote Share Name: FMS plans
Local Mount Directory: mountdir
============================================================
Retrieving flight plan and placing it into /root/xplane_scripts/fetch_simbrief/mountdir
================WGET OUTPUT================
2024-01-13 16:39:26 URL:https://www.simbrief.com/system/briefing.fmsdl.php?formatget=flightplans/KRDUKCLT_XPE_1705153423.fms [320/320] -> "/root/xplane_scripts/fetch_simbrief/mountdir/SB.fms" [1]
===========================================
==============FLIGHT OVERVIEW==============
Release: 2
Icao_airline: AAL
Flight_number: 2964
Is_etops: 0
Dx_rmk: PLANNED OPTIMUM FLIGHT LEVEL
Sys_rmk/:
Is_detailed_profile: 1
Cruise_profile: CI 78
Climb_profile: 250/280/78
Descent_profile: 78/280/250
Alternate_profile: CI0
Reserve_profile: RES
Costindex: 78
Cont_rule: 15 MIN
Initial_altitude: 22000
Stepclimb_string: KRDU/0220
Avg_temp_dev: 6
Avg_tropopause: 50196
Avg_wind_comp: -89
Avg_wind_dir: 240
Avg_wind_spd: 103
Gc_distance: 113
Route_distance: 167
Air_distance: 235
Total_burn: 4869
Cruise_tas: 443
Cruise_mach: .72
Passengers: 183
Route: SHPRD4 CATAR DCT SDAIL CHSLY5
Route_ifps: SHPRD4 CATAR DCT SDAIL CHSLY5
Route_navigraph: SHPRD4 CATAR DCT SDAIL CHSLY5
============================================
Should you wish to file this flight plan with Vatsim, use the following link:
https://my.vatsim.net/pilots/flightplan?raw=%28FPL-AAL2964-IS+-B738%2FM-SDE2E3FGHIRWXY%2FLB1+-KRDU1048+-N0443F220+SHPRD4+CATAR+SDAIL+CHSLY5+-KCLT0045+KATL+-PBN%2FA1B1C1D1S1S2+DOF%2F240113+REG%2FN806SB+EET%2FKZTL0017+OPR%2FAAL+PER%2FC+RMK%2FTCAS+SIMBRIEF%29&fuel_time=0225
What the flight plan looks like
# cat SB.fms
I
1100 Version
CYCLE 2203
ADEP KRDU
DEPRWY RW05L
SID SHPRD4
ADES KCLT
DESRWY RW36R
STAR CHSLY5
STARTRANS SDAIL
NUMENR 4
1 KRDU ADEP 435.000000 35.877639 -78.787472
11 CATAR DRCT 22000.000000 35.835403 -79.300181
11 SDAIL DRCT 22000.000000 35.959100 -79.813883
1 KCLT ADES 3200.000000 35.213750 -80.949056
Note: No, you don’t need to be root…and from a security standpoint, you shouldn’t be :P. However(!) - if you are using the smb mount option, make sure whatever user you are using has the privileges to execute the mount.
Loading the plan
For the Zibo, you can input the flight plan file name (without the .fms extension - “SB” by default) into the CO ROUTE
area of the FMC as shown below. Other planes should also be able to use it, but the process for selecting it will vary by flight management system.
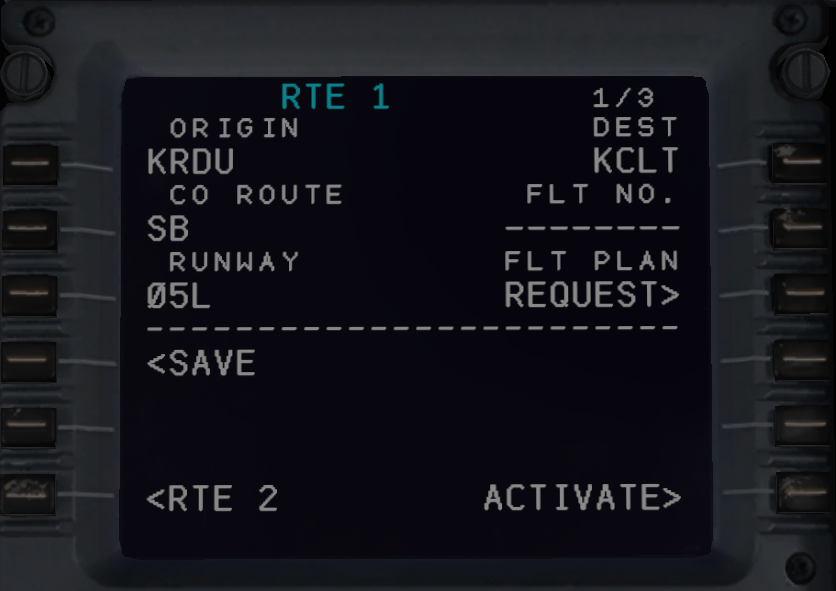
Cheers!